100
|
How can I remove an event
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutAutoSize(EXCALENDARLib::exFixedSize);
spCalendar1->PutFixedCellWidth(36);
spCalendar1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutImage(1);
spCalendar1->GetEvents()->Remove(spCalendar1->GetDate());
|
99
|
How can I count the events
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutImage(1);
long var_Count = spCalendar1->GetEvents()->GetCount();
|
98
|
How can I clear all events
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutImage(1);
spCalendar1->GetEvents()->Clear();
|
96
|
How can I assign an icon to a date
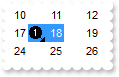
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutAutoSize(EXCALENDARLib::exFixedSize);
spCalendar1->PutFixedCellWidth(36);
spCalendar1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutImage(1);
|
95
|
How can I enable or disable a date

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutDisabled(VARIANT_TRUE);
|
94
|
How can I mark or highlight a date

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutMarker(VARIANT_TRUE);
|
93
|
How can I assign a tooltip or a comment to a date
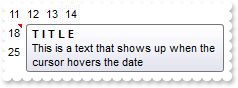
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
EXCALENDARLib::IEventPtr var_Event = spCalendar1->GetEvents()->Add(spCalendar1->GetDate());
var_Event->PutComment(L"This is a text that shows up when the cursor hovers the date");
var_Event->PutCommentTitle(L"T I T L E");
spCalendar1->PutHideSelection(VARIANT_TRUE);
|
92
|
How can I assign any extra data to a date
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutUserData("your data");
|
91
|
How can I change the background color for a date

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutBackColor(RGB(255,0,0));
|
90
|
How can I change the foreground color for a date

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutForeColor(RGB(255,0,0));
|
89
|
How can I draw a date as strikeout

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutStrikeOut(VARIANT_TRUE);
|
88
|
How can I underline a date

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutUnderline(VARIANT_TRUE);
|
87
|
How can I make italic a date

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutItalic(VARIANT_TRUE);
|
86
|
How can I bold a date

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutBold(VARIANT_TRUE);
|
85
|
How can I get the last visible date being displayed in the calendar
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutShowNonMonthDays(VARIANT_FALSE);
spCalendar1->GetEvents()->Add(spCalendar1->GetLastVisibleDate())->PutComment(L"This is the last visible date");
|
84
|
How can I get the first visible date being displayed in the calendar
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutShowNonMonthDays(VARIANT_FALSE);
spCalendar1->GetEvents()->Add(spCalendar1->GetFirstVisibleDate())->PutComment(L"This is the first visible date");
|
83
|
How can I hide the ticker that's shown for dates that have a tooltip or a comment
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutCommentBackColor(spCalendar1->GetBackColor());
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutComment(L"This is a text that shows up when the cursor hovers the date");
|
82
|
How can I change the visual aspect of the dates that have a comment or a tooltip assigned

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spCalendar1->PutCommentBackColor(0x1000000);
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutComment(L"This is a text that shows up when the cursor hovers the date");
spCalendar1->PutHideSelection(VARIANT_TRUE);
|
81
|
How can I change the visual aspect of the dates that have a comment or a tooltip assigned
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
EXCALENDARLib::IAppearancePtr var_Appearance = spCalendar1->GetVisualAppearance();
var_Appearance->Add(11,"c:\\exontrol\\images\\normal.ebn");
var_Appearance->Add(1,"CP:11 4 2 -2 -2");
spCalendar1->PutCommentBackColor(0x1000000);
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutComment(L"This is a text that shows up when the cursor hovers the date");
spCalendar1->PutHideSelection(VARIANT_TRUE);
|
80
|
How can I change the color to highlight the dates that have a comment or a tooltip assigned
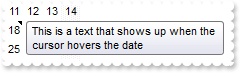
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutCommentBackColor(RGB(0,0,0));
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutComment(L"This is a text that shows up when the cursor hovers the date");
spCalendar1->PutHideSelection(VARIANT_TRUE);
|
79
|
How can I hide the days that are shown in gray, and doesn' belong to the month
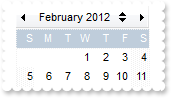
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutShowNonMonthDays(VARIANT_FALSE);
|
78
|
How can I change the color for non working days
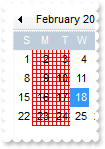
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutNonworkingDays(6);
spCalendar1->PutNonworkingDaysPattern(EXCALENDARLib::exPatternCross);
spCalendar1->PutNonworkingDaysColor(RGB(255,0,0));
|
77
|
How can I change the pattern or the style to draw the non working days
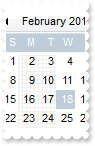
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutNonworkingDays(6);
spCalendar1->PutNonworkingDaysPattern(EXCALENDARLib::exPatternCross);
|
76
|
How can I show or hide the non working days
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutNonworkingDays(0);
|
75
|
How can I specify the non working days
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutNonworkingDays(6);
|
72
|
How can I specify the min and max years to scroll within
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutMinScrollYear(2001);
spCalendar1->PutMaxScrollYear(2010);
|
71
|
How do I let the tooltip being displayed longer
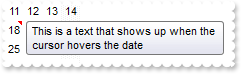
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutToolTipPopDelay(10000);
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutComment(L"This is a text that shows up when the cursor hovers the date");
spCalendar1->PutHideSelection(VARIANT_TRUE);
|
69
|
How can I change the date that gets the focus
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutDate(COleDateTime(2001,1,1,0,00,00).operator DATE());
spCalendar1->PutFocusDate(COleDateTime(2001,1,2,0,00,00).operator DATE());
|
68
|
How can I select a new date
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutDate(COleDateTime(2001,1,1,0,00,00).operator DATE());
spCalendar1->PutValue(COleDateTime(2001,1,2,0,00,00).operator DATE());
|
67
|
I've seen that the width of the tooltip is variable. Can I make it larger
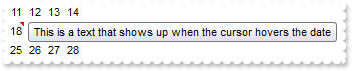
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutToolTipWidth(328);
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutComment(L"This is a text that shows up when the cursor hovers the date");
spCalendar1->PutHideSelection(VARIANT_TRUE);
|
66
|
How do I disable showing the tooltip for all control
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutToolTipDelay(0);
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutComment(L"This is a text that shows up when the cursor hovers the date");
spCalendar1->PutHideSelection(VARIANT_TRUE);
|
65
|
How do I show the tooltip quicker
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutToolTipDelay(1);
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutComment(L"This is a text that shows up when the cursor hovers the date");
spCalendar1->PutHideSelection(VARIANT_TRUE);
|
64
|
How do I call your x-script language
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->ExecuteTemplate(L"BackColor = RGB(255,0,0)");
|
63
|
How do I call your x-script language
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutTemplate(L"BackColor = RGB(255,0,0)");
|
62
|
Can I change the font for the tooltip
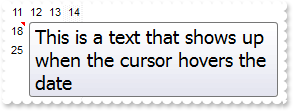
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutToolTipDelay(1);
/*
Copy and paste the following directives to your header file as
it defines the namespace 'stdole' for the library: 'OLE Automation'
#import <stdole2.tlb>
*/
stdole::FontPtr var_StdFont = spCalendar1->GetToolTipFont();
var_StdFont->PutName(L"Tahoma");
var_StdFont->PutSize(_variant_t(long(14)));
spCalendar1->PutToolTipWidth(364);
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutComment(L"This is a text that shows up when the cursor hovers the date");
spCalendar1->PutHideSelection(VARIANT_TRUE);
|
61
|
How can I change the caption of the Today's button
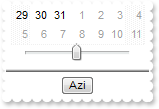
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutTodayCaption(L"Azi");
|
59
|
How do I unselect a date
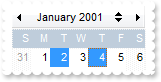
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutSingleSel(VARIANT_FALSE);
spCalendar1->PutDate(COleDateTime(2001,1,1,0,00,00).operator DATE());
spCalendar1->PutSelDate(COleDateTime(2001,1,2,0,00,00).operator DATE());
spCalendar1->PutSelDate(COleDateTime(2001,1,3,0,00,00).operator DATE());
spCalendar1->PutSelDate(COleDateTime(2001,1,4,0,00,00).operator DATE());
spCalendar1->UnSelDate(COleDateTime(2001,1,3,0,00,00).operator DATE());
|
58
|
How do I get the list of selected dates
// SelectionChanged event - Fired when the selection is changed.
void OnSelectionChangedCalendar1()
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
OutputDebugStringW( _bstr_t(spCalendar1->GetSelDate()) );
}
|
57
|
How do I select multiple dates
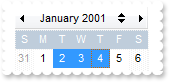
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutSingleSel(VARIANT_FALSE);
spCalendar1->PutDate(COleDateTime(2001,1,1,0,00,00).operator DATE());
spCalendar1->PutSelDate(COleDateTime(2001,1,2,0,00,00).operator DATE());
spCalendar1->PutSelDate(COleDateTime(2001,1,3,0,00,00).operator DATE());
spCalendar1->PutSelDate(COleDateTime(2001,1,4,0,00,00).operator DATE());
|
56
|
How do I get the selected date
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutSelDate(spCalendar1->GetDate());
|
55
|
How can I build a date expression
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutDate(spCalendar1->GetDoDate(2001,1,1));
|
54
|
How can I select a new date
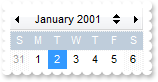
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutDate(spCalendar1->GetDoDate(2001,1,1));
spCalendar1->PutSelDate(spCalendar1->GetDoDate(2001,1,2));
|
53
|
How can I select a new date
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutDate(COleDateTime(2001,1,1,0,00,00).operator DATE());
spCalendar1->PutSelDate(COleDateTime(2001,1,2,0,00,00).operator DATE());
|
52
|
How can I change the browsed date
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutDate(COleDateTime(2001,1,1,0,00,00).operator DATE());
|
51
|
How can I show or hide the buttons that in the header
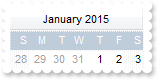
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutShowYearSelector(VARIANT_FALSE);
spCalendar1->PutShowMonthSelector(VARIANT_FALSE);
|
50
|
How can I show or hide the buttons that scrolls the years
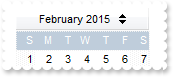
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutShowYearSelector(VARIANT_FALSE);
|
49
|
How can I disable changing the month in the months selector
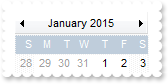
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutShowMonthSelector(VARIANT_FALSE);
|
48
|
How can I change the visual aspect of the selected date, using your EBN

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutBackColor(RGB(255,255,255));
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spCalendar1->PutSelBackColor(0x1000000);
spCalendar1->PutSelForeColor(spCalendar1->GetBackColor());
|
47
|
How can I change the colors for selected dates

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutSelBackColor(RGB(0,0,0));
spCalendar1->PutSelForeColor(RGB(255,255,255));
|
46
|
How can I change the color of the line arround a month
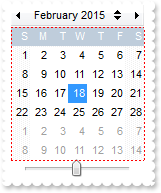
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutDrawBorderLine(EXCALENDARLib::LargeDots);
spCalendar1->PutBorderLineColor(RGB(255,0,0));
|
45
|
How can I change the style of the line arround a month
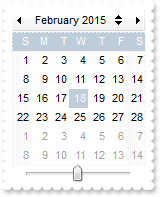
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutDrawBorderLine(EXCALENDARLib::LargeDots);
|
44
|
How can I specify the number of months being displayed
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutShowTodayButton(VARIANT_FALSE);
spCalendar1->PutShowYearScroll(VARIANT_FALSE);
spCalendar1->PutMinMonthX(2);
spCalendar1->PutMaxMonthX(2);
spCalendar1->PutMinMonthY(2);
spCalendar1->PutMaxMonthY(2);
|
43
|
How can I lock the control, so user can't select a new date
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutLocked(VARIANT_TRUE);
|
42
|
How do I change the first day of the week
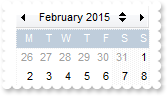
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutFirstDay(EXCALENDARLib::Monday);
|
41
|
How do I change the name of the months
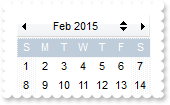
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutMonthNames(L"Jan Feb Mar Apr May Jun Jul Aug Sep Oct Nov Dec");
|
40
|
How do I change the name of the months
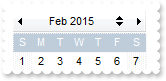
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutMonthName(EXCALENDARLib::January,L"Jan");
spCalendar1->PutMonthName(EXCALENDARLib::February,L"Feb");
spCalendar1->PutMonthName(EXCALENDARLib::March,L"Mar");
spCalendar1->PutMonthName(EXCALENDARLib::April,L"Apr");
spCalendar1->PutMonthName(EXCALENDARLib::May,L"May");
spCalendar1->PutMonthName(EXCALENDARLib::June,L"Jun");
spCalendar1->PutMonthName(EXCALENDARLib::July,L"Jul");
spCalendar1->PutMonthName(EXCALENDARLib::August,L"Aug");
spCalendar1->PutMonthName(EXCALENDARLib::September,L"Sep");
spCalendar1->PutMonthName(EXCALENDARLib::October,L"Oct");
spCalendar1->PutMonthName(EXCALENDARLib::November,L"Nov");
spCalendar1->PutMonthName(EXCALENDARLib::December,L"Dec");
|
39
|
How do I change the name for each week day
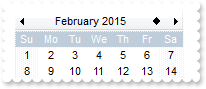
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutAutoSize(EXCALENDARLib::exFixedSize);
spCalendar1->PutFixedCellWidth(24);
spCalendar1->PutFixedCellHeight(16);
spCalendar1->PutWeekDays(L"Su Mo Tu We Th Fr Sa");
spCalendar1->PutHeaderForeColor(RGB(255,0,0));
spCalendar1->PutHeaderBackColor(RGB(255,255,255));
|
38
|
How can I specify the width and height for dates being displayed in the calendar
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutAutoSize(EXCALENDARLib::exFixedSize);
spCalendar1->PutFixedCellWidth(22);
spCalendar1->PutFixedCellHeight(16);
|
37
|
How can I specify the width and height for dates being displayed in the calendar
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutAutoSize(EXCALENDARLib::exFixedSize);
spCalendar1->PutFixedCellWidth(32);
spCalendar1->PutFixedCellHeight(24);
|
36
|
How do I change the appearance for dates in the calendar
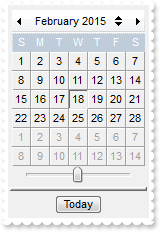
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutAppearanceDay(EXCALENDARLib::Day3D);
spCalendar1->PutBackColor(0x8000000f);
|
35
|
How do I specify the color for the grid lines
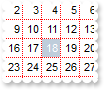
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutDrawGridLine(EXCALENDARLib::SmallDots);
spCalendar1->PutGridLineColor(RGB(255,0,0));
|
34
|
How do I draw the grid lines
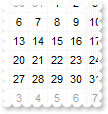
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutDrawGridLine(EXCALENDARLib::SmallDots);
|
33
|
How do I change the foreground color for the week days and week numbers header
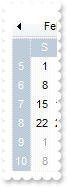
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutHeaderForeColor(RGB(255,0,0));
spCalendar1->PutShowWeeks(VARIANT_TRUE);
|
32
|
How do I change the background color for the week days and week numbers header
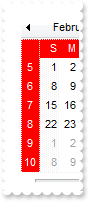
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutHeaderBackColor(RGB(255,0,0));
spCalendar1->PutShowWeeks(VARIANT_TRUE);
|
31
|
How can I show or hide the 'Today' button
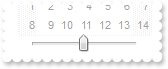
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutShowTodayButton(VARIANT_FALSE);
|
30
|
How can I show or hide the scroll bar that changes the year
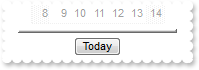
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutShowYearScroll(VARIANT_FALSE);
|
29
|
How can I show or hide the header that displays the months
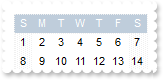
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutShowMonth(VARIANT_FALSE);
|
28
|
How can I show or hide the header that displays the week days
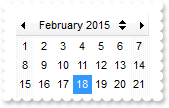
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutShowDays(VARIANT_FALSE);
|
27
|
How do I show or hide the weeks
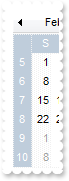
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutShowWeeks(VARIANT_TRUE);
|
26
|
How can I change the visual appearance of Today date in the calendar

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutMarkToday(VARIANT_TRUE);
spCalendar1->PutBackground(EXCALENDARLib::exMarkToday,RGB(255,0,0));
|
25
|
How do I mark the Today date

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutMarkToday(VARIANT_TRUE);
|
24
|
How can I show the control's selection even if the control loses the focus

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutHideSelection(VARIANT_FALSE);
|
23
|
How can I hide the control's selection when the control loses the focus

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutHideSelection(VARIANT_TRUE);
|
22
|
How do I enable single selection
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutSingleSel(VARIANT_TRUE);
|
21
|
How do I enable multiple selection
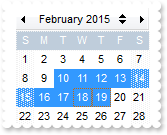
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutSingleSel(VARIANT_FALSE);
|
20
|
How do I disable or enable the control
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutEnabled(VARIANT_FALSE);
|
19
|
Can I change the forecolor for the tooltip
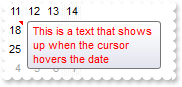
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutToolTipDelay(1);
spCalendar1->PutToolTipWidth(364);
spCalendar1->PutBackground(EXCALENDARLib::exToolTipForeColor,RGB(255,0,0));
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutComment(L"This is a text that shows up when the cursor hovers the date");
spCalendar1->PutHideSelection(VARIANT_TRUE);
|
18
|
Can I change the background color for the tooltip
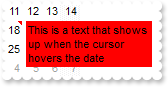
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutToolTipDelay(1);
spCalendar1->PutToolTipWidth(364);
spCalendar1->PutBackground(EXCALENDARLib::exToolTipBackColor,RGB(255,0,0));
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutComment(L"This is a text that shows up when the cursor hovers the date");
spCalendar1->PutHideSelection(VARIANT_TRUE);
|
17
|
Can I change the default border of the tooltip, using your EBN files
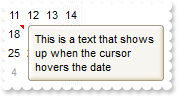
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutToolTipDelay(1);
spCalendar1->PutToolTipWidth(364);
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spCalendar1->PutBackground(EXCALENDARLib::exToolTipAppearance,0x1000000);
spCalendar1->GetEvents()->Add(spCalendar1->GetDate())->PutComment(L"This is a text that shows up when the cursor hovers the date");
spCalendar1->PutHideSelection(VARIANT_TRUE);
|
16
|
How can I change the visual appearance of the selected month, in the months selector, using EBN files
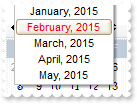
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spCalendar1->PutBackground(EXCALENDARLib::exMonthSelect,0x1000000);
spCalendar1->PutBackground(EXCALENDARLib::exMonthSelectForeColor,RGB(255,0,0));
|
15
|
How can I change the visual appearance of Today date in the calendar, using EBN files
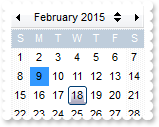
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\pushed.ebn");
spCalendar1->PutBackground(EXCALENDARLib::exMarkToday,0x1000000);
spCalendar1->PutBackground(EXCALENDARLib::exDropDownSelForeColor,RGB(1,0,0));
spCalendar1->PutSelForeColor(RGB(0,0,0));
spCalendar1->PutMarkToday(VARIANT_TRUE);
|
14
|
How can I change the visual appearance of separator between calendar and 'Today' button , using EBN files
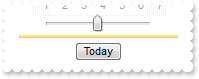
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spCalendar1->PutBackground(EXCALENDARLib::exDateSeparatorBar,0x1000000);
|
13
|
How can I change the visual appearance of the scrolling bar for years, using EBN files
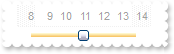
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spCalendar1->GetVisualAppearance()->Add(2,"c:\\exontrol\\images\\pushed.ebn");
spCalendar1->PutBackground(EXCALENDARLib::exDateScrollRange,0x1000000);
spCalendar1->PutBackground(EXCALENDARLib::exDateScrollThumb,0x2000000);
|
12
|
How can I change the visual appearance of 'Today' button, using EBN files
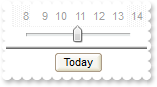
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spCalendar1->GetVisualAppearance()->Add(2,"c:\\exontrol\\images\\pushed.ebn");
spCalendar1->PutBackground(EXCALENDARLib::exDateTodayUp,0x1000000);
spCalendar1->PutBackground(EXCALENDARLib::exDateTodayDown,0x2000000);
|
11
|
How can I change the visual appearance of header , using EBN files
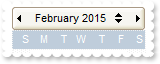
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spCalendar1->PutBackground(EXCALENDARLib::exDateHeader,0x1000000);
spCalendar1->PutHeaderForeColor(RGB(255,0,0));
|
10
|
How can I change the visual appearance of header that week numbers, using EBN files
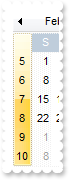
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spCalendar1->PutBackground(EXCALENDARLib::exWeeksHeader,0x1000000);
spCalendar1->PutHeaderForeColor(RGB(255,0,0));
spCalendar1->PutShowWeeks(VARIANT_TRUE);
|
9
|
How can I change the visual appearance of header that displays days, using EBN files
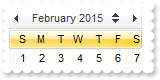
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spCalendar1->PutBackground(EXCALENDARLib::exDaysHeader,0x1000000);
|
8
|
How can I change the visual appearance of the drop down button, using EBN files
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spCalendar1->GetVisualAppearance()->Add(2,"c:\\exontrol\\images\\pushed.ebn");
spCalendar1->PutBackground(EXCALENDARLib::exDropDownButtonUp,0x1000000);
spCalendar1->PutBackground(EXCALENDARLib::exDropDownButtonDown,0x2000000);
|
7
|
How do I change the visual appearance of the left and right buttons in the calendar's header, using EBN files
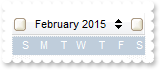
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spCalendar1->PutBackground(EXCALENDARLib::exScrollLeft,0x1000000);
spCalendar1->PutBackground(EXCALENDARLib::exScrollRight,0x1000000);
|
6
|
How do I change the visual appearance of the up and down buttons in the calendar's header, using EBN files
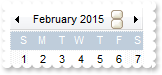
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spCalendar1->PutBackground(EXCALENDARLib::exScrollUp,0x1000000);
spCalendar1->PutBackground(EXCALENDARLib::exScrollDown,0x1000000);
|
5
|
How do I change the control's foreground color
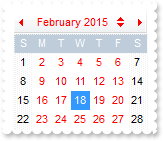
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutForeColor(RGB(255,0,0));
|
4
|
How do I change the control's background color
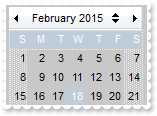
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutBackColor(RGB(200,200,200));
|
3
|
How can I change the control's font
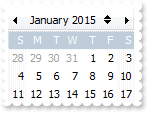
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetFont()->PutName(L"Verdana");
|
2
|
How do I change the control's border, using your EBN files
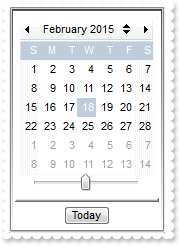
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spCalendar1->PutAppearance(EXCALENDARLib::AppearanceEnum(0x1000000));
|
1
|
How do I remove the control's border
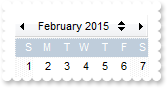
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCALENDARLib' for the library: 'ExCalendar 1.0 Control Library'
#import <ExCalendar.dll>
using namespace EXCALENDARLib;
*/
EXCALENDARLib::ICalendarPtr spCalendar1 = GetDlgItem(IDC_CALENDAR1)->GetControlUnknown();
spCalendar1->PutAppearance(EXCALENDARLib::None2);
|